基础数据类型(set集合)
认识集合
由一个或多个确定的元素所构成的整体叫做集合。
集合中的元素有三个特征:
1.确定性(集合中的元素必须是确定的)
2.互异性(集合中的元素互不相同。例如:集合A={1,a},则a不能等于1)
3.无序性(集合中的元素没有先后之分),如集合{3,4,5}和{3,5,4}算作同一个集合。
*集合概念存在的目的是将不同的值存放到一起,不同的集合间用来做关系运算,无需纠结于集合中某个值
集合的定义
s = {1,2,3,1}
#定义可变集合>>> set_test=set('hello')
>>> set_test
{'l', 'o', 'e', 'h'}#改为不可变集合frozenset>>> f_set_test=frozenset(set_test)
>>> f_set_test
frozenset({'l', 'e', 'h', 'o'})
集合的常用操作及关系运算
元素的增加
单个元素的增加 : add(),add的作用类似列表中的append
对序列的增加 : update(),而update类似extend方法,update方法可以支持同时传入多个参数:
>>> a={1,2}
>>> a.update([3,4],[1,2,7])
>>> a
{1, 2, 3, 4, 7}
>>> a.update("hello")
>>> a
{1, 2, 3, 4, 7, 'h', 'e', 'l', 'o'}
>>> a.add("hello")
>>> a
{1, 2, 3, 4, 'hello', 7, 'h', 'e', 'l', 'o'}
元素的删除
集合删除单个元素有两种方法:
元素不在原集合中时:
set.discard(x)不会抛出异常
set.remove(x)会抛出KeyError错误
>>> a={1,2,3,4}
>>> a.discard(1)
>>> a
{2, 3, 4}
>>> a.discard(1)
>>> a
{2, 3, 4}
>>> a.remove(1)
Traceback (most recent call last):
File "<input>", line 1, in <module>
KeyError: 1
pop():由于集合是无序的,pop返回的结果不能确定,且当集合为空时调用pop会抛出KeyError错误,
clear():清空集合
>>> a={3,"a",2.1,1}
>>> a.pop()
1
>>> a.pop()
3
>>> a.clear()
>>> a
set()
>>> a.pop()
Traceback (most recent call last):
File "<input>", line 1, in <module>
KeyError: 'pop from an empty set'
集合操作
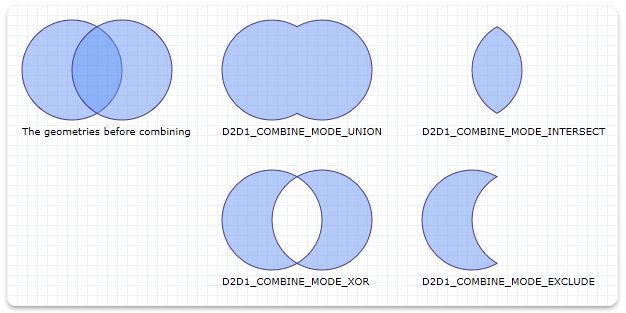
|,|=:合集
a = {1,2,3}
b = {2,3,4,5}print(a.union(b))print(a|b)
&.&=:交集
a = {1,2,3}
b = {2,3,4,5}print(a.intersection(b))print(a&b)
-,-=:差集
a = {1,2,3}
b = {2,3,4,5}print(a.difference(b))print(a-b)
^,^=:对称差集
a = {1,2,3}
b = {2,3,4,5}print(a.symmetric_difference(b))print(a^b)
包含关系
in,not in:判断某元素是否在集合内
==,!=:判断两个集合是否相等
两个集合之间一般有三种关系,相交、包含、不相交。在Python中分别用下面的方法判断:
set.isdisjoint(s):判断两个集合是不是不相交
set.issuperset(s):判断集合是不是包含其他集合,等同于a>=b
set.issubset(s):判断集合是不是被其他集合包含,等同于a<=b
集合的工厂函数
class set(object):
"""
set() -> new empty set object
set(iterable) -> new set object
Build an unordered collection of unique elements.
""" def add(self, *args, **kwargs): # real signature unknown """
Add an element to a set.
This has no effect if the element is already present.
""" pass def clear(self, *args, **kwargs): # real signature unknown """ Remove all elements from this set. """ pass def copy(self, *args, **kwargs): # real signature unknown """ Return a shallow copy of a set. """ pass def difference(self, *args, **kwargs): # real signature unknown """
相当于s1-s2
Return the difference of two or more sets as a new set.
(i.e. all elements that are in this set but not the others.)
""" pass def difference_update(self, *args, **kwargs): # real signature unknown """ Remove all elements of another set from this set. """ pass def discard(self, *args, **kwargs): # real signature unknown """
与remove功能相同,删除元素不存在时不会抛出异常
Remove an element from a set if it is a member.
If the element is not a member, do nothing.
""" pass def intersection(self, *args, **kwargs): # real signature unknown """
相当于s1&s2
Return the intersection of two sets as a new set.
(i.e. all elements that are in both sets.)
""" pass def intersection_update(self, *args, **kwargs): # real signature unknown """ Update a set with the intersection of itself and another. """ pass def isdisjoint(self, *args, **kwargs): # real signature unknown """ Return True if two sets have a null intersection. """ pass def issubset(self, *args, **kwargs): # real signature unknown """
相当于s1<=s2
Report whether another set contains this set. """ pass def issuperset(self, *args, **kwargs): # real signature unknown """
相当于s1>=s2
Report whether this set contains another set. """ pass def pop(self, *args, **kwargs): # real signature unknown """
Remove and return an arbitrary set element.
Raises KeyError if the set is empty.
""" pass def remove(self, *args, **kwargs): # real signature unknown """
Remove an element from a set; it must be a member.
If the element is not a member, raise a KeyError.
""" pass def symmetric_difference(self, *args, **kwargs): # real signature unknown """
相当于s1^s2
Return the symmetric difference of two sets as a new set.
(i.e. all elements that are in exactly one of the sets.)
""" pass def symmetric_difference_update(self, *args, **kwargs): # real signature unknown """ Update a set with the symmetric difference of itself and another. """ pass def union(self, *args, **kwargs): # real signature unknown """
相当于s1|s2
Return the union of sets as a new set.
(i.e. all elements that are in either set.)
""" pass def update(self, *args, **kwargs): # real signature unknown """ Update a set with the union of itself and others. """ pass def __and__(self, *args, **kwargs): # real signature unknown """ Return self&value. """ pass def __contains__(self, y): # real signature unknown; restored from __doc__ """ x.__contains__(y) <==> y in x. """ pass def __eq__(self, *args, **kwargs): # real signature unknown """ Return self==value. """ pass def __getattribute__(self, *args, **kwargs): # real signature unknown """ Return getattr(self, name). """ pass def __ge__(self, *args, **kwargs): # real signature unknown """ Return self>=value. """ pass def __gt__(self, *args, **kwargs): # real signature unknown """ Return self>value. """ pass def __iand__(self, *args, **kwargs): # real signature unknown """ Return self&=value. """ pass def __init__(self, seq=()): # known special case of set.__init__ """
set() -> new empty set object
set(iterable) -> new set object
Build an unordered collection of unique elements.
# (copied from class doc)
""" pass def __ior__(self, *args, **kwargs): # real signature unknown """ Return self|=value. """ pass def __isub__(self, *args, **kwargs): # real signature unknown """ Return self-=value. """ pass def __iter__(self, *args, **kwargs): # real signature unknown """ Implement iter(self). """ pass def __ixor__(self, *args, **kwargs): # real signature unknown """ Return self^=value. """ pass def __len__(self, *args, **kwargs): # real signature unknown """ Return len(self). """ pass def __le__(self, *args, **kwargs): # real signature unknown """ Return self<=value. """ pass def __lt__(self, *args, **kwargs): # real signature unknown """ Return self<value. """ pass @staticmethod # known case of __new__ def __new__(*args, **kwargs): # real signature unknown """ Create and return a new object. See help(type) for accurate signature. """ pass def __ne__(self, *args, **kwargs): # real signature unknown """ Return self!=value. """ pass def __or__(self, *args, **kwargs): # real signature unknown """ Return self|value. """ pass def __rand__(self, *args, **kwargs): # real signature unknown """ Return value&self. """ pass def __reduce__(self, *args, **kwargs): # real signature unknown """ Return state information for pickling. """ pass def __repr__(self, *args, **kwargs): # real signature unknown """ Return repr(self). """ pass def __ror__(self, *args, **kwargs): # real signature unknown """ Return value|self. """ pass def __rsub__(self, *args, **kwargs): # real signature unknown """ Return value-self. """ pass def __rxor__(self, *args, **kwargs): # real signature unknown """ Return value^self. """ pass def __sizeof__(self): # real signature unknown; restored from __doc__ """ S.__sizeof__() -> size of S in memory, in bytes """ pass def __sub__(self, *args, **kwargs): # real signature unknown """ Return self-value. """ pass def __xor__(self, *args, **kwargs): # real signature unknown """ Return self^value. """ pass __hash__ = None