一、组合模式简介(Brief Introduction)
组合模式,将对象组合成树形结构以表示“部分
-
整体”的层次结构,组合模式使得用户对单个对象和组合对象的使用具有一致性。
二、解决的问题(What To Solve)
解决整合与部分可以被一致对待问题。
三、组合模式分析(Analysis)
1、组合模式结构
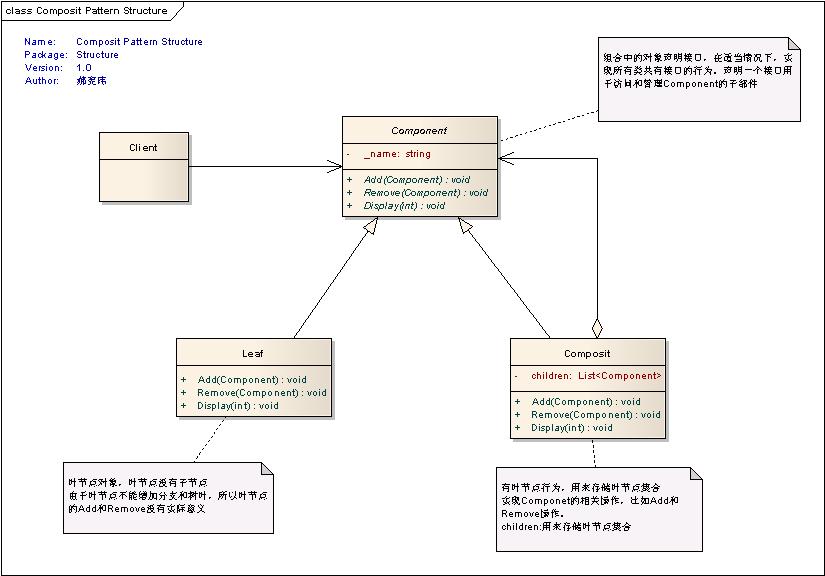
Component
类:组合中的对象声明接口,在适当情况下,实现所有类共有接口的行为。声明一个接口用于访问和管理
Component
的子部件
Leaf
类:叶节点对象,叶节点没有子节点。由于叶节点不能增加分支和树叶,所以叶节点的
Add
和
Remove
没有实际意义。
有叶节点行为,用来存储叶节点集合
Composite
类:实现
Componet
的相关操作,比如
Add
和
Remove
操作。
children:
用来存储叶节点集合
2、源代码
1
、抽象类
Component
|
public
abstract class Component
{
protected string
name;
public
Component(string
name)
{
this
.name = name;
}
public abstract void
Add(Component
c);
public abstract void
Remove(Component
c);
public abstract void
Diaplay(int
depth);
}
|
2
、叶子节点
Leaf
继承于
Component
|
public
class Leaf
:Component
{
public
Leaf(string
name)
:base
(name)
{
}
public override void
Add(Component
c)
{
Console
.WriteLine("
不能向叶子节点添加子节点"
);
}
public override void
Remove(Component
c)
{
Console
.WriteLine("
叶子节点没有子节点"
);
}
public override void
Diaplay(int
depth)
{
Console
.WriteLine(new string
('-'
,depth)+name);
}
}
|
3
、组合类
Composite
继承于
Component
,
拥有枝节点行为
|
public
class Composite
: Component
{
List
<Component
> children;
public
Composite(string
name)
:base
(name)
{
if
(children == null
)
{
children = new List
<Component
>();
}
}
public override void
Add(Component
c)
{
this
.children.Add(c);
}
public override void
Remove(Component
c)
{
this
.children.Remove(c);
}
public override void
Diaplay(int
depth)
{
Console
.WriteLine(new String
('-'
,depth)+name);
foreach
(Component
component in
children)
{
component.Diaplay(depth + 2);
}
}
}
|
4
、客户端代码
|
static
void Main
(string
[] args)
{
Composite
root = new Composite
("
根节点root"
);
root.Add(new Leaf
("
根上生出的叶子A"
));
root.Add(new Leaf
("
根上生出的叶子B"
));
Composite
comp = new Composite
("
根上生出的分支CompositeX"
);
comp.Add(new Leaf
("
分支CompositeX
生出的叶子LeafXA"
));
comp.Add(new Leaf
("
分支CompositeX
生出的叶子LeafXB"
));
root.Add(comp);
Composite
comp2 = new Composite
("
分支CompositeX
生出的分支CompositeXY"
);
comp2.Add(new Leaf
("
分支CompositeXY
生出叶子LeafXYA"
));
comp2.Add(new Leaf
("
分支CompositeXY
生出叶子LeafXYB"
));
comp.Add(comp2);
root.Add(new Leaf
("
根节点生成的叶子LeafC"
));
Leaf
leafD = new Leaf
("leaf D"
);
root.Add(leafD);
root.Remove(leafD);
root.Diaplay(1);
Console
.Read();
}
|
3、程序运行结果
本文转自 灵动生活 51CTO博客,原文链接:http://blog.51cto.com/smartlife/267502,如需转载请自行联系原作者